MoneyWorks Manual
Ask (controlType, name, value, definition, …)
Result type: array
Definition: The Ask function provides a simple custom UI for data capture. It takes a variable number of parameters that define the custom controls to appear in the dialog box. The general form is:
ControlTypes are “static”, “text”, “password”, “number”, “date”, “checkbox”, “popup”, “radio”, “title” and “invis”. These are case-sensitive strings. If you use any other string for controlType, that will be taken as the name (i.e. content) for a static text control. The text, password, number, date, radio, and checkbox types have a name and a value (static just has a name). The popup type has a name, a value, and a definition. The title type has a single value, which will be used as the window title. The function knows how many following parameters to expect after each controltype parameter.
The buttons are always OK and Cancel.
Example:
let result = Ask("This is a prompt", "number", "Number of Coins", 5, "checkbox", "They are Gold", 0, "title", "My big ask")
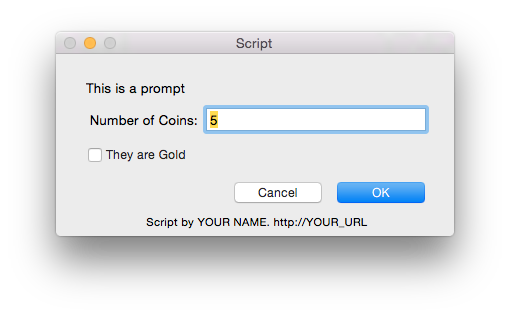
The function result is an associative array whose keys are the control names (with spaces replaced by underscores, in the same manner as custom controls for reports). There is also a key-value with key "ok" and value 0 or 1 denoting whether the OK button was clicked.
e.g. result["Choose_one"] → "bar"
result["Number_of_Coins"] → 5
result["They_are_Gold"] → 0
To implement validation handlers for the Ask dialog box, you must pass an invisible control with the name "ident", thus:
... "invis", "ident", "com_yourdomain_My_Ask"
This defines an invisible control with the special name "ident" which becomes the dialog box's symbolic identifier for the purposes of message interception.
IMPORTANT: Since every Ask dialog will be different, but all loaded scripts receive UI messages for dialogs, the handlers for validating them must be unique across all scripts that could possibly be installed in the same document). You MUST choose a globally unique identifier (do not use the one in this example). It is recommended that you use the reverse domain name convention to derive a globally unique name. For example, I would use names in the form nz_co_cognito_rowan_myscript_ask. This should provide reasonable assurance that no-one else's scripts will try to trap messages for the ask dialog in my script.
Keep in mind the 63-character limit on handler names.
Example:
on Load let a = Ask("text","Some Text","Default","invis","ident", "nz_co_cognito_MyAsk") if a["OK"] alert(a["Some_Text"]) else say("cancelled") endif end on ValidateField:nz_co_cognito_MyAsk:Some_text(winref, fieldID, value) say(value) end
Note: Ask() parameters will be truncated to 255 characters. To include a long menu, use AppendPopupItems in the Before handler of the Ask dialog. For example, the following will display a menu of available invoice forms:
on Before:unique_MyAsk(w) let formlist = "None;(-;"+replace(GetPlugIns("forms", "all", "invc"), "\n", ";") AppendPopupItems(w, "Invoice", formlist) end on Load let result = Ask("Choose Form", "popup", "Invoice", "", "", "invis","ident", "unique_MyAsk") end
Availability: within an MWScript handler
See Also:
Alert: Display an alert with up to 3 buttons
ChooseFromList: Very simple list dialog box
Say: Speak some text